Simple Browser Application tutorial
1.Introduction
In this tutorial we are going to create a simple browser. This browser will have a UIWebView to load the different pages, a UISearchBar that will search the web via Google and present the results on UIWebView and a UIToolbar for back, forward, and home buttons.
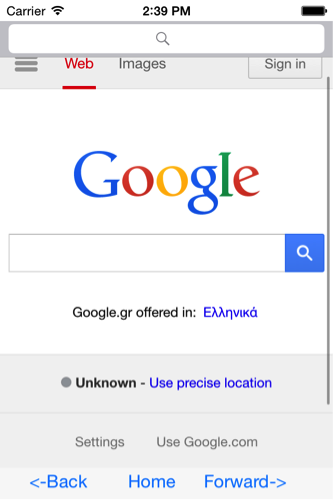
2.Adding the UIWebview
First we are going to add the UIWebView. The View will take full width and cover the screen but we have to make sure that we leave enough space for the search bar and the toolbar. Particularly we will need 20px for the device bar (the one with the signal, time and battery on top.), 35px for the search bar and 30px for the toolbar placed at the bottom of the screen containing the buttons Back, Home and Forward. So the code that defines all these specifications is the following:
final UIWebView webview = new UIWebView(new CGRect(0, 55, dWidth, dHeight - 30 - 20 - 35));
webview.setScalesPageToFit(true);
webview.loadRequest(new NSURLRequest(NSURL.URLWithString("http://www.google.com")));
webview.scrollView();
(dHeight and dWidth are the devices height and width, for more information on UIView placement look at the UIView tutorial.)
Next we set scalePagesToFit property to true, so that the pages viewed will be scaled in order to fit. Also we call the scrollview method so that me make the view scrollable.
Finally we load our url with loadRequest method:
webview.loadRequest(new NSURLRequest(NSURL.URLWithString(“http://www.google.com”)));.
If you want to load a different page replace the string parameter with the URL of the page you want. (Be sure that you use the whole URL path i.e. http://….).
In case you need to load a page from a local file use the NSURL.fileURLWithPath(path) method instead.
3.Adding the search bar
The next step is to add our search bar. We are going to use the UISearchBar class that does not actually perform any searches but implements a text field control for texted-based searches.
So we are going to create an object of that class which will go at the top of the screen just below the phone info bar (the one with the signal icon). Its distance from the top will be 20px and its height will be 35px.Here’s the respective code:
UISearchBar search = new UISearchBar(new CGRect(0, 20, dWidth, 35));
search.setDelegate(new UISearchBarDelegate() {
@Override
public void searchSearchButtonClicked(UISearchBar p1) {
String searchstring = p1.text().replaceAll(" ", "+");
webview.loadRequest(new NSURLRequest(NSURL.URLWithString("https://www.google.gr/search?q=" + searchstring)));
}
});
For the needs of this tutorial we will set the delegate to perform a Google search when the search button is clicked. To do that we override the searchSearchButtonClicked(UISearchBar p1) method to call webView’s loadReaquest with the google searchstring(“https://www.google.gr/search?q=”) plus the user input. (Don’t forget that the webview loads a URL so we have to replace all spaces with ”+” first).
4.Adding the toolbar
We add the toolbar 30px above the bottom with 30px height and full width.
UIToolbar toolbar = new UIToolbar(new CGRect(0, dHeight - 30, dWidth, 30));
UIBarButtonItem back = new UIBarButtonItem("<-Back", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() { @Override public void clicked() { webview.goBack(); } }); back.setWidth(dWidth / 4); UIBarButtonItem home = new UIBarButtonItem("Home", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() { @Override public void clicked() { webview.loadRequest(new NSURLRequest(NSURL.URLWithString("http://www.google.com"))); } }); home.setWidth(dWidth / 4); UIBarButtonItem forward = new UIBarButtonItem("Forward->", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() {
@Override
public void clicked() {
webview.goForward();
}
});
forward.setWidth(dWidth / 4);
List barButtons = new ArrayList();
barButtons.add(back);
barButtons.add(home);
barButtons.add(forward);
toolbar.setItems(barButtons, true);
toolbar.setBarStyle(UIBarStyle.Default);
After that we create the toolbar buttons. In this tutorial we initialize the UIBarButtonItems with a title, a style and their delegate. (You can also initialize them with images or system button variables).
In each button we set the delegate to do the buttons action when clicked.
We add the Buttons to a list and that list to the toolbar with the toolbar.setItems(barButtons,true) method.
5.Finally
We need to add everything above to the main View:
6.The whole code
Main.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.simplebrowser;
import crossmobile.ios.uikit.UIApplication;
public class Main {
public static void main(String[] args) {
UIApplication.main(args, null, ApplicationDelegate.class);
}
}
ApplicationDelegate.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.simplebrowser;
import crossmobile.ios.uikit.UIApplication;
import crossmobile.ios.uikit.UIApplicationDelegate;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UIWindow;
import java.util.Map;
public class ApplicationDelegate implements UIApplicationDelegate {
private UIWindow window;
@Override
public boolean didFinishLaunchingWithOptions(UIApplication app, Map<String, Object> launchOptions) {
window = new UIWindow(UIScreen.mainScreen().bounds());
window.setRootViewController(new ViewController());
window.makeKeyAndVisible();
return true;
}
}
ViewController.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.simplebrowser;
import crossmobile.ios.coregraphics.CGRect;
import crossmobile.ios.foundation.NSURL;
import crossmobile.ios.foundation.NSURLRequest;
import crossmobile.ios.uikit.UIBarButtonItem;
import crossmobile.ios.uikit.UIBarButtonItemDelegate;
import crossmobile.ios.uikit.UIBarButtonItemStyle;
import crossmobile.ios.uikit.UIBarStyle;
import crossmobile.ios.uikit.UIColor;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UISearchBar;
import crossmobile.ios.uikit.UISearchBarDelegate;
import crossmobile.ios.uikit.UIToolbar;
import crossmobile.ios.uikit.UIView;
import crossmobile.ios.uikit.UIViewController;
import crossmobile.ios.uikit.UIWebView;
import java.util.ArrayList;
import java.util.List;
public class ViewController extends UIViewController {
float dHeight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
@Override
public void loadView() {
UIView mainView = new UIView();
mainView.setBackgroundColor(UIColor.whiteColor());
final UIWebView webview = new UIWebView(new CGRect(0, 55, dWidth, dHeight - 30 - 20 - 35));
webview.setScalesPageToFit(true);
webview.loadRequest(new NSURLRequest(NSURL.URLWithString("http://www.google.com")));
webview.scrollView();
UISearchBar search = new UISearchBar(new CGRect(0, 20, dWidth, 35));
search.setDelegate(new UISearchBarDelegate() {
@Override
public void searchButtonClicked(UISearchBar p1) {
String searchstring = p1.text().replaceAll(" ", "+");
webview.loadRequest(new NSURLRequest(NSURL.URLWithString("https://www.google.gr/search?q=" + searchstring)));
}
});
UIToolbar toolbar = new UIToolbar(new CGRect(0, dHeight - 30, dWidth, 30));
UIBarButtonItem back = new UIBarButtonItem("<-Back", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() { @Override public void clicked() { webview.goBack(); } }); back.setWidth(dWidth / 4); UIBarButtonItem home = new UIBarButtonItem("Home", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() { @Override public void clicked() { webview.loadRequest(new NSURLRequest(NSURL.URLWithString("http://www.google.com"))); } }); home.setWidth(dWidth / 4); UIBarButtonItem forward = new UIBarButtonItem("Forward->", UIBarButtonItemStyle.Bordered, new UIBarButtonItemDelegate() {
@Override
public void clicked() {
webview.goForward();
}
});
forward.setWidth(dWidth / 4);
List barButtons = new ArrayList();
barButtons.add(back);
barButtons.add(home);
barButtons.add(forward);
toolbar.setItems(barButtons, true);
toolbar.setBarStyle(UIBarStyle.Default);
mainView.addSubview(toolbar);
mainView.addSubview(search);
mainView.addSubview(webview);
setView(mainView);
}
}