Truth or Dare application tutorial
In this tutorial we will make a Truth or Dare game. We are going to use landscape orientation, custom image for background, custom buttons and JSON parsing.
1.Creating the project – choosing Landscape view
First of all open CrossMobile and click New Project
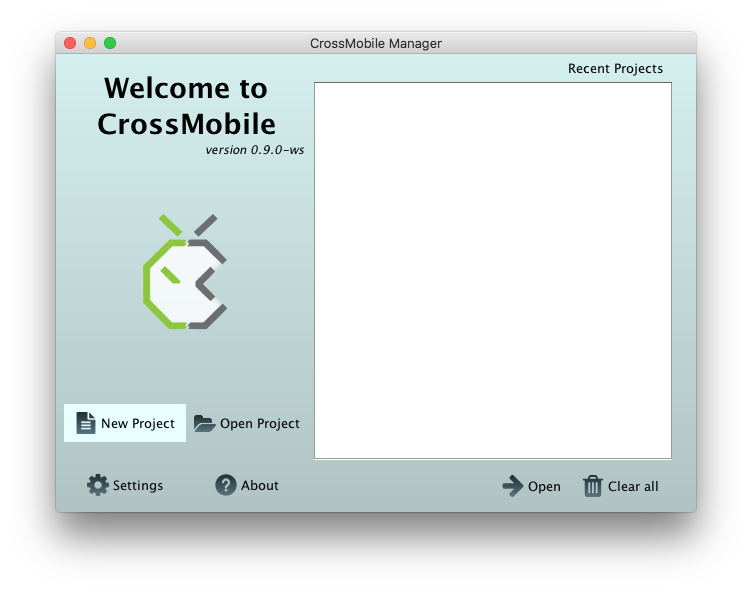
Name your Project TruthOrDare and press Create:
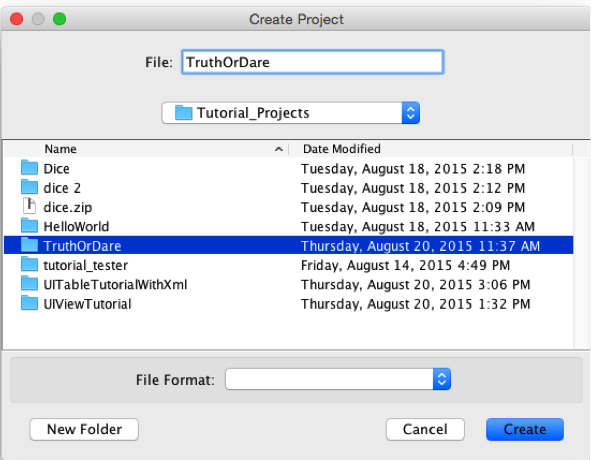
Go to the visuals tab, select “Left” for Initial Orientation and choose only “Left” on Supported Orientations.
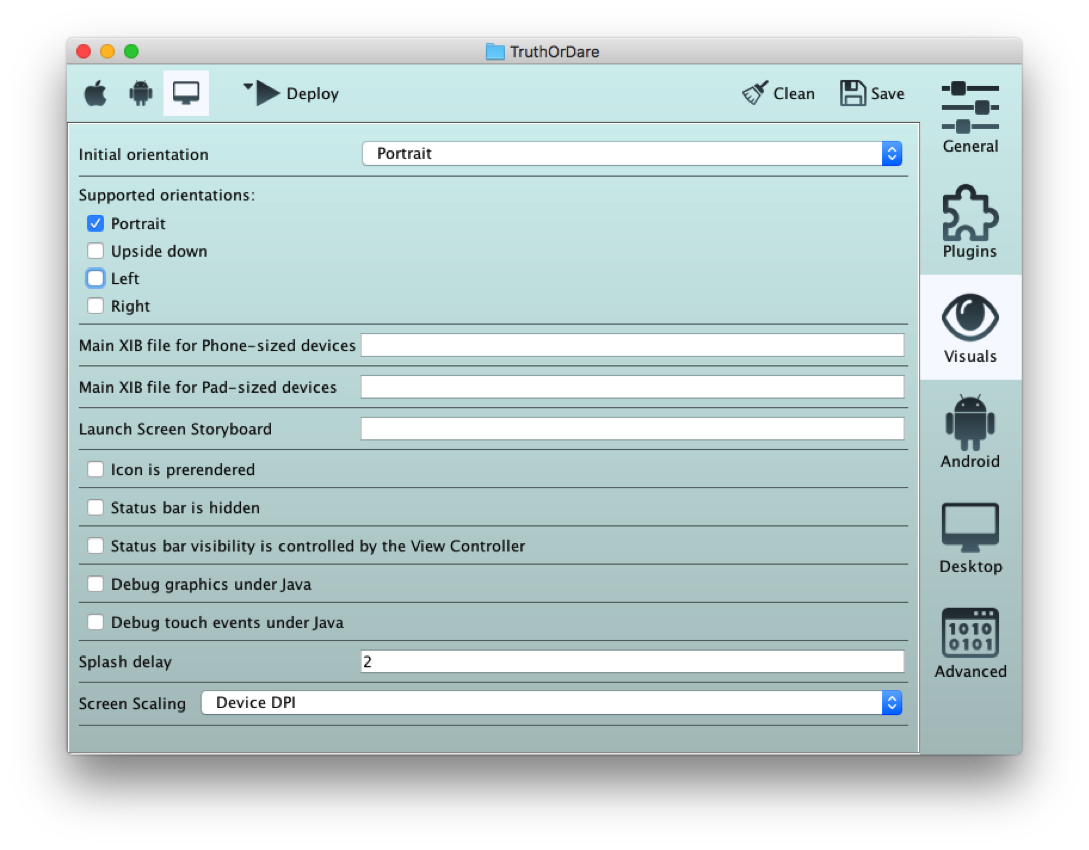
Now we need to make our questions. To do that we will use JSON format. JSON (JavaScript Object Notation) is an interchange format intended to be machine and human readable. JSON is based on two elements:
- A collection of name/value pairs
- An ordered list of values
So our JSON file would be like this:
{
"truth": [
"Who was your first crush, or who is your current crush?",
"How old were you when you had your first kiss?",
"What would you do if your current boyfriend/girlfriend ended things right now?",
],
"dare": [
"Go outside and sing a clip of your favorite Disney song at the top of your lungs.",
"Exchange shirts with the person next to you for the next round of questions.",
"Wear a funny hat on your head for the next three rounds of questions.",
]
}
This example contains three truths and three dares. You can change and/or add questions, just keep the format. (A more complete json file can be downloaded from here: questions.json
Create your questions with the above format and save it as questions.json in the project folder src/main/artwork.
Then download artwork.zip and extract the pictures in the artwork subfolder in same directory. (be careful to add the pictures directly in the artwork folder and not in their own subfolder or the device will not find them)
Finally select desktop icon and Code to open the project with NetBeans.
2.Starting the project
Add a UINavigationController to ApplicationDelegate.java to support more than one UIViewControllers (we need three for this project)
public class ApplicationDelegate implements UIApplicationDelegate {
private UIWindow window;
@Override
public boolean didFinishLaunchingWithOptions(UIApplication app, Map<String, Object> launchOptions) {UIWindow window = new UIWindow(UIScreen.mainScreen().bounds());
UINavigationController navControl = new UINavigationController(new ViewController());
navControl.setNavigationBarHidden(true);
window.setRootViewController(navControl);
window.makeKeyAndVisible();
return true;
}
}
Go to ViewController
1public class ViewController extends UIViewController {
2
3 @Override
4 public void loadView() {
5 UIView mainView = new UIView();
6 mainView.setBackgroundColor(UIColor.whiteColor());
7
8 UILabel title = new UILabel(new CGRect(0, 390, 320, 40));
9 title.setText("Hello World!");
10 title.setTextAlignment(UITextAlignment.Center);
11
12 UIImageView img = new UIImageView(new CGRect(75, 80, 170, 220));
13 img.setImage(UIImage.imageNamed("logo.png"));
14
15 mainView.addSubview(title);
16 mainView.addSubview(img);
17
18 setView(mainView);
19 }
20}
And delete everything between lines 7 and 17.
1public class ViewController extends UIViewController {
2
3 @Override
4 public void loadView() {
5 UIView mainView = new UIView();
6 mainView.setBackgroundColor(UIColor.whiteColor());
7
8 setView(mainView);
9 }
10}
First we need to add two fields to dynamically allocate the Views
public class ViewController extends UIViewController {
float dheight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
These two fields represent the devices width and height. (To see more about dynamically allocating views go to UIView – CGRect Tutorial
To add a picture as background create a UIImageView that takes the whole screen and add it to the mainView first. Since everything else will be added after that view they will be on top of it. (for more information on handling Views look here).
UIImage img = UIImage.imageNamed("background.png");
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setImage(img);
background.setContentMode(UIViewContentMode.ScaleToFill);
//add first so it remains on the background
mainView.addSubview(background);
Also set Content Mode to ScaleToFill for the image scale to your device’s dimensions.
Then we will add a Custom button that starts the game and a label that informs it.
UIButton startBTN = UIButton.buttonWithType(UIButtonType.Custom);
startBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
startBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
startBTN.setTitle("Start Game", UIControlState.Normal);
startBTN.setTitleColor(UIColor.colorWithHSBA(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
startBTN.setFrame(new CGRect(dWidth / 2 - 200, dheight/2 + 30, 400, 60));
startBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new GameControler(), true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(title);
mainView.addSubview(startBTN);
This time we are going to add an image for the Button, or , to be more precise , two, with the setBackgroundImage(UIImage.imageNamed(“button_normal”), UIControlState.Normal); and setBackgroundImage(UIImage.imageNamed(“button_highlighted”), UIControlState.Highlighted);.
This simply means that when the button is pressed it will change its image from button_normal to button_highlighted, that gives the button a more natural button pressing feel, it also gives the user a response that its working.
Also we will use a custom color for the button tittle, setTitleColor(UIColor.colorWithHSBA(0.55f, 0.47f, 0.57f, 1) in this case we use HSBA (Hue, Saturation , Brightness and Alpha).
Note that we set HSBA with floats representing percentages. (so Hue that takes values from 0 to 360 has to converted to float)
Don’t forget to add the Views on the main view or they will not show.
(For more information on buttons and labels go to the Dice tutorial or the CrossMobile Documentation)
3.Creating the GameController
In this UIViewController we add the background a descriptive label that informs the buttons and to buttons that push the TruthOrDareController initialized accordingly. The TruthOrDareController takes a string as parameter that specifies the kind of question it should search for, “truth” or “dare”.
public class GameControler extends UIViewController {
float dheight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
@Override
public void loadView() {
UIView mainView = new UIView();
UIImage img = UIImage.imageNamed("background.png");
mainView.setBackgroundColor(UIColor.whiteColor());
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setImage(img);
background.setContentMode(UIViewContentMode.ScaleToFill);
UILabel title = new UILabel(new CGRect(0, dheight/2 - 20, dWidth, 40));
title.setText("Choose Truth or Dare");
title.setTextAlignment(UITextAlignment.Center);
UIButton truthBTN = UIButton.buttonWithType(UIButtonType.Custom);
truthBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
truthBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
truthBTN.setTitle("Truth", UIControlState.Normal);
truthBTN.setTitleColor(UIColor.colorWithHSBA(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
truthBTN.setFrame(new CGRect(dWidth / 2 - 165, dheight/2 + 30, 150, 60));
truthBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new TruthOrDareController("truth"), true);
}
}, UIControlEvents.TouchDown);
UIButton dareBTN = UIButton.buttonWithType(UIButtonType.Custom);
dareBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
dareBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
dareBTN.setTitle("Dare", UIControlState.Normal);
dareBTN.setTitleColor(UIColor.colorWithHSBA(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
dareBTN.setFrame(new CGRect(dWidth / 2 + 15, dheight/2 + 30, 150, 60));
dareBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new TruthOrDareController("dare"), true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(background);
mainView.addSubview(title);
mainView.addSubview(truthBTN);
mainView.addSubview(dareBTN);
setView(mainView);
}
}
4.Creating the truth or dare controller
float dheight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
private final String mode;
private final Random random;
public TruthOrDareController(String mode) {
this.mode = mode;
random = new Random();
}
First we create the two fields needed for width and height, a String field for setting the game mode, and a random so we can get a random question later.
Then we add the background and the Button to go back so we can choose the next question, leaving enough space to add the UITextView.
@Override
public void loadView() {
UIView mainView = new UIView();
UIImage img = UIImage.imageNamed("background.png");
mainView.setBackgroundColor(UIColor.whiteColor());
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setImage(img);
background.setContentMode(UIViewContentMode.ScaleToFill);
mainView.addSubview(background);
. . . . . . . . . . . . . . . . .
UIButton startBTN = UIButton.buttonWithType(UIButtonType.Custom);
startBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
startBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
startBTN.setTitle("Next", UIControlState.Normal);
startBTN.setTitleColor(UIColor.colorWithHSBA(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
startBTN.setFrame(new CGRect(dWidth / 2 - 200, dheight/2 + 60, 400, 60));
startBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, int arg1) {
navigationController().popViewControllerAnimated(true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(question);
mainView.addSubview(startBTN);
setView(mainView);
}
}
5.Adding the UITextView and parsing Json
First we are going to parse the truth or dare questions, depending on the parameter, and then add a UITextView to add the question to mainView
String path = NSBundle.mainBundle().pathForResource("questions", "json");
NSData data = NSData.dataWithContentsOfFile(path);
Map<String,Object> jsonObject = (Map<String,Object>) NSJSONSerialization.JSONObjectWithData(data,0, null);
List array = (List) jsonObject.get(mode);
UITextView question = new UITextView(new CGRect(0, dheight/2 - 50, dWidth, 100));
question.setEditable(false);
question.setBackgroundColor(UIColor.clearColor());
question.setFont(UIFont.systemFontOfSize(24));
question.setText( array.get(random.nextInt(array.size())));
question.setTextAlignment(UITextAlignment.Center);
To add the UITextView we will handle it as any view, initialize with CGRect and set it s attributes.
In this case we set editable to false since there is no need to edit the questions, backgroundColor to clearColor since we have our background, made the texts font a little bigger, alignment to center and the text as a random question from our list.
Don’t forget to add your views to mainView or they will not show.
6.The whole code
Main.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.truthordare;
import crossmobile.ios.uikit.UIApplication;
public class Main {
public static void main(String[] args) {
UIApplication.main(args, null, ApplicationDelegate.class);
}
}
ApplicationDelegate.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.truthordare;
import crossmobile.ios.uikit.UIApplication;
import crossmobile.ios.uikit.UIApplicationDelegate;
import crossmobile.ios.uikit.UINavigationController;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UIWindow;
import java.util.Map;
public class ApplicationDelegate implements UIApplicationDelegate {
private UIWindow window;
@Override
public boolean didFinishLaunchingWithOptions(UIApplication app, Map<String, Object> launchOptions) {UIWindow window = new UIWindow(UIScreen.mainScreen().bounds());
UINavigationController navControl = new UINavigationController(new ViewController());
navControl.setNavigationBarHidden(true);
window.setRootViewController(navControl);
window.makeKeyAndVisible();
return true;
}
}
ViewController.java
/*
* This project was created with CrossMobile library.
* More info at http://www.crossmobile.org
*/
package my.company.truthordare;
import crossmobile.ios.coregraphics.CGRect;
import crossmobile.ios.uikit.UIButton;
import crossmobile.ios.uikit.UIButtonType;
import crossmobile.ios.uikit.UIColor;
import crossmobile.ios.uikit.UIControl;
import crossmobile.ios.uikit.UIControlDelegate;
import crossmobile.ios.uikit.UIControlEvents;
import crossmobile.ios.uikit.UIControlState;
import crossmobile.ios.uikit.UIEvent;
import crossmobile.ios.uikit.UIImage;
import crossmobile.ios.uikit.UIImageView;
import crossmobile.ios.uikit.UILabel;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UITextAlignment;
import crossmobile.ios.uikit.UIView;
import crossmobile.ios.uikit.UIViewContentMode;
import crossmobile.ios.uikit.UIViewController;
public class ViewController extends UIViewController {
float dheight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
@Override
public void loadView() {
UIView mainView = new UIView();
UIImage img = UIImage.imageNamed("background.png");
mainView.setBackgroundColor(UIColor.whiteColor());
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setImage(img);
background.setContentMode(UIViewContentMode.ScaleToFill); //add first so it remains on the background mainView.addSubview(background);
UILabel title = new UILabel(new CGRect(0, dheight / 2 - 20, dWidth, 40));
title.setText("Press Start");
title.setTextAlignment(UITextAlignment.Center);
UIButton startBTN = UIButton.buttonWithType(UIButtonType.Custom);
startBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
startBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
startBTN.setTitle("Start Game", UIControlState.Normal);
startBTN.setTitleColor(UIColor.colorWithHueSaturationBrightnessAlpha(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
startBTN.setFrame(new CGRect(dWidth / 2 - 200, dheight / 2 + 30, 400, 60));
startBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new GameController(), true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(title);
mainView.addSubview(startBTN);
setView(mainView);
}
}
GameController.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package my.company.truthordare;
import crossmobile.ios.coregraphics.CGRect;
import crossmobile.ios.uikit.UIButton;
import crossmobile.ios.uikit.UIButtonType;
import crossmobile.ios.uikit.UIColor;
import crossmobile.ios.uikit.UIControl;
import crossmobile.ios.uikit.UIControlDelegate;
import crossmobile.ios.uikit.UIControlEvents;
import crossmobile.ios.uikit.UIControlState;
import crossmobile.ios.uikit.UIEvent;
import crossmobile.ios.uikit.UIImage;
import crossmobile.ios.uikit.UIImageView;
import crossmobile.ios.uikit.UILabel;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UITextAlignment;
import crossmobile.ios.uikit.UIView;
import crossmobile.ios.uikit.UIViewContentMode;
import crossmobile.ios.uikit.UIViewController;
public class GameController extends UIViewController{
float dWidth = UIScreen.mainScreen().bounds().size.width;
float dheight = UIScreen.mainScreen().bounds().size.height;
@Override
public void loadView() {
UIView mainView = new UIView();
UIImage img = UIImage.imageNamed("background.png");
mainView.setBackgroundColor(UIColor.whiteColor());
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setImage(img);
background.setContentMode(UIViewContentMode.ScaleToFill);
UILabel title = new UILabel(new CGRect(0, dheight / 2 - 20, dWidth, 40));
title.setText("Choose Truth or Dare");
title.setTextAlignment(UITextAlignment.Center);
UIButton truthBTN = UIButton.buttonWithType(UIButtonType.Custom);
truthBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
truthBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
truthBTN.setTitle("Truth", UIControlState.Normal);
truthBTN.setTitleColor(UIColor.colorWithHueSaturationBrightnessAlpha(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
truthBTN.setFrame(new CGRect(dWidth / 2 - 165, dheight / 2 + 30, 150, 60));
truthBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new TruthOrDareController("truth"), true);
}
}, UIControlEvents.TouchDown);
UIButton dareBTN = UIButton.buttonWithType(UIButtonType.Custom);
dareBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
dareBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
dareBTN.setTitle("Dare", UIControlState.Normal);
dareBTN.setTitleColor(UIColor.colorWithHueSaturationBrightnessAlpha(0.55f, 0.47f, 0.57f, 1),UIControlState.Normal);
dareBTN.setFrame(new CGRect(dWidth / 2 + 15, dheight / 2 + 30, 150, 60));
dareBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().pushViewController(new TruthOrDareController("dare"), true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(background);
mainView.addSubview(title);
mainView.addSubview(truthBTN);
mainView.addSubview(dareBTN);
setView(mainView);
}
}
TruthOrDareController.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package my.company.truthordare;
import crossmobile.ios.coregraphics.CGRect;
import crossmobile.ios.foundation.NSBundle;
import crossmobile.ios.foundation.NSData;
import crossmobile.ios.foundation.NSJSONSerialization;
import crossmobile.ios.uikit.UIButton;
import crossmobile.ios.uikit.UIButtonType;
import crossmobile.ios.uikit.UIColor;
import crossmobile.ios.uikit.UIControl;
import crossmobile.ios.uikit.UIControlDelegate;
import crossmobile.ios.uikit.UIControlEvents;
import crossmobile.ios.uikit.UIControlState;
import crossmobile.ios.uikit.UIEvent;
import crossmobile.ios.uikit.UIFont;
import crossmobile.ios.uikit.UIImage;
import crossmobile.ios.uikit.UIImageView;
import crossmobile.ios.uikit.UIScreen;
import crossmobile.ios.uikit.UITextAlignment;
import crossmobile.ios.uikit.UITextView;
import crossmobile.ios.uikit.UIView;
import crossmobile.ios.uikit.UIViewContentMode;
import crossmobile.ios.uikit.UIViewController;
import java.util.List;
import java.util.Map;
import java.util.Random;
public class TruthOrDareController extends UIViewController{
float dheight = UIScreen.mainScreen().bounds().size.height;
float dWidth = UIScreen.mainScreen().bounds().size.width;
private final String mode;
private final Random random;
public TruthOrDareController(String mode) {
this.mode = mode;
random = new Random();
}
@Override
public void loadView() {
UIView mainView = new UIView();
UIImage img = UIImage.imageNamed("background.png");
mainView.setBackgroundColor(UIColor.whiteColor());
UIImageView background = new UIImageView(new CGRect(0, 0, dWidth, dheight));
background.setContentMode(UIViewContentMode.ScaleToFill);
mainView.addSubview(background);
String path = NSBundle.mainBundle().pathForResource("questions","json");
NSData data = NSData.dataWithContentsOfFile(path);
Map<String, Object> jsonObject = (Map<String, Object>) NSJSONSerialization.JSONObjectWithData(data, 0, null);
List array = (List) jsonObject.get(mode);
UITextView question = new UITextView(new CGRect(0, dheight / 2 - 50, dWidth, 100));
question.setEditable(false);
question.setBackgroundColor(UIColor.clearColor());
question.setFont(UIFont.systemFontOfSize(24));
question.setText(array.get(random.nextInt(array.size())));
question.setTextAlignment(UITextAlignment.Center);
UIButton startBTN = UIButton.buttonWithType(UIButtonType.Custom);
startBTN.setBackgroundImage(UIImage.imageNamed("button_normal"), UIControlState.Normal);
startBTN.setBackgroundImage(UIImage.imageNamed("button_highlighted"), UIControlState.Highlighted);
startBTN.setTitle("Next", UIControlState.Normal);
startBTN.setTitleColor(UIColor.colorWithHueSaturationBrightnessAlpha(0.55f, 0.47f, 0.57f, 1), UIControlState.Normal);
startBTN.setFrame(new CGRect(dWidth / 2 - 200, dheight / 2 + 60, 400, 60));
startBTN.addTarget(new UIControlDelegate() {
@Override
public void exec(UIControl arg0, UIEvent arg1) {
navigationController().popViewControllerAnimated(true);
}
}, UIControlEvents.TouchDown);
mainView.addSubview(question);
mainView.addSubview(startBTN);
setView(mainView);
}
}
questions.json
{
"truth": [
"Who was your first crush, or who is your current crush?",
"How old were you when you had your first kiss?",
"What would you do if your current boyfriend/girlfriend ended things right now?",
"Have you ever cheated on a boyfriend/girlfriend?",
"Have you ever been cheated on by a boyfriend/girlfriend?",
"If you could go on a date with anyone in the room, who would it be?",
"What personality traits would cause you to end a friendship?",
"Would you go behind a friend's back with a crush?",
"Have you ever lied to your best friend?",
"Would you ever cheat off a friends paper?",
"How many different best friends have you had during your lifetime?",
"If you were stuck on a deserted island, which friend would you want with you?",
"How long have you gone without showering?",
"Have you ever told a lie during a game of Truth or Dare? What was it and why?",
"Have you ever had a crush on anyone here?",
"Have you ever stolen anything?",
"What's your scariest nightmare?",
"What do you think is your biggest physical flaw?",
"Have you ever gone skinny dipping?",
"What kind of pajamas do you wear to bed?",
"What's the dumbest thing you've ever done on a dare?",
"What color is your underwear?",
"Have you ever peed in the swimming pool?",
"If you weren't here, what would you be doing?",
"If you couldn't go to the college or get the job of your dreams, what would you do?",
"What is one thing you've never told anyone else?",
"What do you want to be when you grow up?",
"What kind of person do you want to marry someday?",
"Do you want to have kids? How many?",
"If you could switch places with someone for a day, who would it be?",
"If you could invent anything, what would it be?",
"If you knew the world was about to end, what would you do?",
"If you could be born again, who would you come back as?",
"Are you scared of dying? Why?",
"What is your biggest fear?",
"What happened on worst day of your life?",
"Have you ever climbed a tree?",
"Have you ever sang and danced in the grocery store?",
"If you could be a superhero, what would your power be?",
"What would you do if you were invisible for a day?",
"If you life were made into a movie, who would play you?",
"What's your biggest pet peeve?",
"What is your special talent?",
"What's the best meal you've ever had?",
"What's you favorite Disney movie and why?",
"What would you do with a million dollars if you ever won the lottery?"
],
"dare": [
"Go outside and sing a clip of your favorite Disney song at the top of your lungs.",
"Exchange shirts with the person next to you for the next round of questions.",
"Wear a funny hat on your head for the next three rounds of questions.",
"Drink a mystery brew concocted by the rest of the group. Make sure there is nothing harmful or dangerous in the concoction, and set areasonable limit of sips the person must take to complete the dare.",
"Everything you say for the rest of the game has to rhyme.",
"Give someone in the group a piggyback ride around the room.",
"Pretend that you're swimming underwater for the next three rounds of questions.",
"Prank call someone you know (perhaps another girl in the group that couldn't make it that night).",
"Set up a tea party between any of the stuffed animals in the house. Invite the girls in your group to come join.",
"Eat a mouthful of crackers and try to whistle.",
"If there is a pet at the event, have that person try and hold the pet for the rest of the night.",
"Repeat everything another player says for the next three rounds of the game.",
"Wear your pants backward for the rest of the game.",
"Ask the neighbors to borrow a cup of sugar.",
"Sing instead of speaking for the next two rounds of the game.",
"Post a YouTube video after singing a currently popular song.",
"Make up a rap about the person to your right.",
"Run around the room imitating a monkey.",
"Say the alphabet backwards in a British accent.",
"Crack an egg on your head."
]
}